RandomAffine in PyTorch (1)
Buy Me a Coffee☕ *Memos: My post explains RandomAffine() with scale argument. My post explains RandomRotation(). My post explains RandomPerspective(). My post explains OxfordIIITPet(). RandomAffine() can do random rotation or random affine transformation for an image as shown below: *Memos: The 1st argument for initialization is degrees(Required-Type:int, float or tuple/list(int or float)): *Memos: It can do rotation. It's the range of the degrees [min, max] so it must min
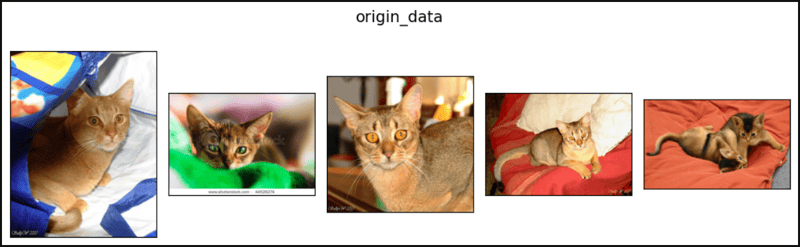
*Memos:
-
My post explains RandomAffine() with
scale
argument. - My post explains RandomRotation().
- My post explains RandomPerspective().
- My post explains OxfordIIITPet().
RandomAffine() can do random rotation or random affine transformation for an image as shown below:
*Memos:
- The 1st argument for initialization is
degrees
(Required-Type:int
,float
ortuple
/list
(int
orfloat
)): *Memos:- It can do rotation.
- It's the range of the degrees
[min, max]
so it mustmin <= max
. - A degrees value is randomly taken from the range of
[min, max]
. - A tuple/list must be the 1D with 2 elements.
- A single value(
int
orfloat
) means[-degrees(min), +degrees(max)]
. - A single value(
int
orfloat
) must be0 <= x
.
- The 2nd argument for initialization is
translate
(Optional-Default:None
-Type:tuple
/list
(int
orfloat
)): *Memos:- It's
[a, b]
. - It must be
0 <= 1
. - It must be the 1D with 2 elements.
- The 1st element is for the horizontal shift randomly taken in the range of
-img_width * a < horizontal shift < img_width * a
. - The 2nd element is for the vertical shift randomly taken in the range of
-img_height * b < vertical shift < img_height * b
.
- It's
- The 3rd argument for initialization is
scale
(Optional-Default:None
-Type:tuple
/list
(int
orfloat
)): *Memos:- It's
[min, max]
so it mustmin <= max
. - It must be
0 < x
. - It must be the 1D with 2 elements.
- A scale value is randomly taken from the range of
[min, max]
.
- It's
- The 4th argument for initialization is
shear
(Optional-Default:None
-Type:int
,float
ortuple
/list
(int
orfloat
)): *Memos:- It can do affine transformation with
x
andy
. - It's
[min, max, min, max]
so it mustmin <= max
. *Memos: - The 1st two elements are the range of
x
. - The 2nd two elements are the range of
y
. -
x
value is randomly taken from the range of the 1st two elements. -
y
value is randomly taken from the range of the 2nd two elements. - A tuple/list must be the 1D with 2 or 4 elements.
- The tuple/list of 2 elements means
[shear[0](min), shear[1](max), 0.0(min), 0.0(min)]
. - A single value means
[-shear(min), +shear(max), 0.0(min), 0.0(max)]
. - A single value must be
0 <= x
.
- It can do affine transformation with
- The 5th argument for initialization is
interpolation
(Optional-Default:InterpolationMode.NEAREST
-Type:InterpolationMode). - The 6th argument for initialization is
fill
(Optional-Default:0
-Type:int
,float
ortuple
/list
(int
orfloat
)): *Memos:- It can change the background of an image. *The background can be seen when doing rotation or affine transformation for an image.
- A tuple/list must be the 1D with 1 or 3 elements.
- The 7th argument for initialization is
center
(Optional-Default:None
-Type:tuple
/list
(int
orfloat
)): *Memos:- It can change the center position of an image.
- It must be the 1D with 2 elements.
- The 1st argument is
img
(Required-Type:PIL Image
ortensor
(int
)): *Memos:- A tensor must be 3D.
- Don't use
img=
.
-
v2
is recommended to use according to V1 or V2? Which one should I use?.
from torchvision.datasets import OxfordIIITPet
from torchvision.transforms.v2 import RandomAffine
from torchvision.transforms.functional import InterpolationMode
randomaffine = RandomAffine(degrees=90)
randomaffine = RandomAffine(degrees=[-90, 90],
translate=None,
scale=None,
shear=None,
interpolation=InterpolationMode.NEAREST,
fill=0,
center=None)
randomaffine
# RandomAffine(degrees=[-90, 90],
# interpolation=InterpolationMode.NEAREST,
# fill=0)
randomaffine.degrees
# [-90.0, 90.0]
print(randomaffine.translate)
# None
print(randomaffine.scale)
# None
print(randomaffine.shear)
# None
randomaffine.interpolation
#
randomaffine.fill
# 0
print(randomaffine.center)
# None
origin_data = OxfordIIITPet(
root="data",
transform=None
# transform=RandomAffine(degrees=[0, 0])
)
d90_data = OxfordIIITPet( # `d` is degrees.
root="data",
transform=RandomAffine(degrees=90)
# transform=RandomAffine(degrees=[-90, 90])
)
dn90_0_data = OxfordIIITPet( # `n` is negative.
root="data",
transform=RandomAffine(degrees=[-90, 0])
)
d0_90_data = OxfordIIITPet(
root="data",
transform=RandomAffine(degrees=[0, 90])
)
dn90n90_data = OxfordIIITPet(
root="data",
transform=RandomAffine(degrees=[-90, -90])
)
d90_90_data = OxfordIIITPet(
root="data",
transform=RandomAffine(degrees=[90, 90])
)
d180_180_data = OxfordIIITPet(
root="data",
transform=RandomAffine(degrees=[180, 180])
)
hrvrtran_data = OxfordIIITPet( # `hr` is horizontal and `vr` is vertical.
root="data", # `tran` is translate.
transform=RandomAffine(degrees=[0, 0], translate=[0.8, 0.5])
)
hrtran_data = OxfordIIITPet(
root="data",
transform=RandomAffine(degrees=[0, 0], translate=[0.8, 0])
)
vrtran_data = OxfordIIITPet(
root="data",
transform=RandomAffine(degrees=[0, 0], translate=[0, 0.5])
)
dn45n45fgray_data = OxfordIIITPet(
root="data",
transform=RandomAffine(degrees=[-45, -45], fill=150)
)
d135_135fpurple_data = OxfordIIITPet(
root="data",
transform=RandomAffine(degrees=[135, 135], fill=[160, 32, 240])
)
d180_180c270_200_data = OxfordIIITPet( # `c` is center.
root="data",
transform=RandomAffine(degrees=[180, 180], center=[270, 200])
)
import matplotlib.pyplot as plt
def show_images1(data, main_title=None):
plt.figure(figsize=[10, 5])
plt.suptitle(t=main_title, y=0.8, fontsize=14)
for i, (im, _) in zip(range(1, 6), data):
plt.subplot(1, 5, i)
plt.imshow(X=im)
plt.xticks(ticks=[])
plt.yticks(ticks=[])
plt.tight_layout()
plt.show()
show_images1(data=origin_data, main_title="origin_data")
print()
show_images1(data=d90_data, main_title="d90_data")
show_images1(data=dn90_0_data, main_title="dn90_0_data")
show_images1(data=d0_90_data, main_title="d0_90_data")
print()
show_images1(data=dn90n90_data, main_title="dn90n90_data")
show_images1(data=d90_90_data, main_title="d90_90_data")
show_images1(data=d180_180_data, main_title="d180_180_data")
print()
show_images1(data=hrvrtran_data, main_title="hrvrtran_data")
show_images1(data=hrtran_data, main_title="hrtran_data")
show_images1(data=vrtran_data, main_title="vrtran_data")
print()
show_images1(data=dn45n45fgray_data, main_title="dn45n45fgray_data")
show_images1(data=d135_135fpurple_data, main_title="d135_135fpurple_data")
show_images1(data=d180_180c270_200_data, main_title="d180_180c270_200_data")
# ↓ ↓ ↓ ↓ ↓ ↓ The code below is identical to the code above. ↓ ↓ ↓ ↓ ↓ ↓
def show_images2(data, main_title=None, d=0, t=None, sc=None, sh=None,
ip=InterpolationMode.NEAREST, f=0, c=None):
plt.figure(figsize=[10, 5])
plt.suptitle(t=main_title, y=0.8, fontsize=14)
for i, (im, _) in zip(range(1, 6), data):
plt.subplot(1, 5, i)
ra = RandomAffine(degrees=d, translate=t, scale=sc, # Here
shear=sh, interpolation=ip, center=c, fill=f)
plt.imshow(X=ra(im)) # Here
plt.xticks(ticks=[])
plt.yticks(ticks=[])
plt.tight_layout()
plt.show()
show_images2(data=origin_data, main_title="origin_data")
print()
show_images2(data=origin_data, main_title="d90_data", d=90)
show_images2(data=origin_data, main_title="dn90_0_data", d=[-90, 0])
show_images2(data=origin_data, main_title="d0_90_data", d=[0, 90])
print()
show_images2(data=origin_data, main_title="dn90n90_data", d=[-90, -90])
show_images2(data=origin_data, main_title="d90_90_data", d=[90, 90])
show_images2(data=origin_data, main_title="d180_180_data", d=[180, 180])
print()
show_images2(data=origin_data, main_title="hrvrtran_data", d=[0, 0],
t=[0.8, 0.5])
show_images2(data=origin_data, main_title="hrtran_data", d=[0, 0],
t=[0.8, 0])
show_images2(data=origin_data, main_title="vrtran_data", d=[0, 0],
t=[0, 0.5])
print()
show_images2(data=origin_data, main_title="dn45n45fgray_data",
d=[-45, -45], f=150)
show_images2(data=origin_data, main_title="d135_135fpurple_data",
d=[135, 135], f=[160, 32, 240])
show_images2(data=origin_data, main_title="d180_180c270_200_data",
d=[180, 180], c=[270, 200])